Short Course Description
OpenMP (Open Multi-Processing) is a widely-used API for shared memory parallel programming, enabling developers to write efficient and scalable parallel applications for multi-core processors. This course is designed to introduce the fundamentals of OpenMP programming, providing skills to leverage shared memory architectures effectively. Through a combination of theoretical sessions and hands-on exercises, participants will learn how to parallelize their applications using OpenMP directives, work-sharing constructs, synchronization mechanisms, and performance optimization techniques. Whether you are new to parallel programming or looking to enhance your expertise, this course offers a comprehensive introduction to OpenMP for shared memory systems.
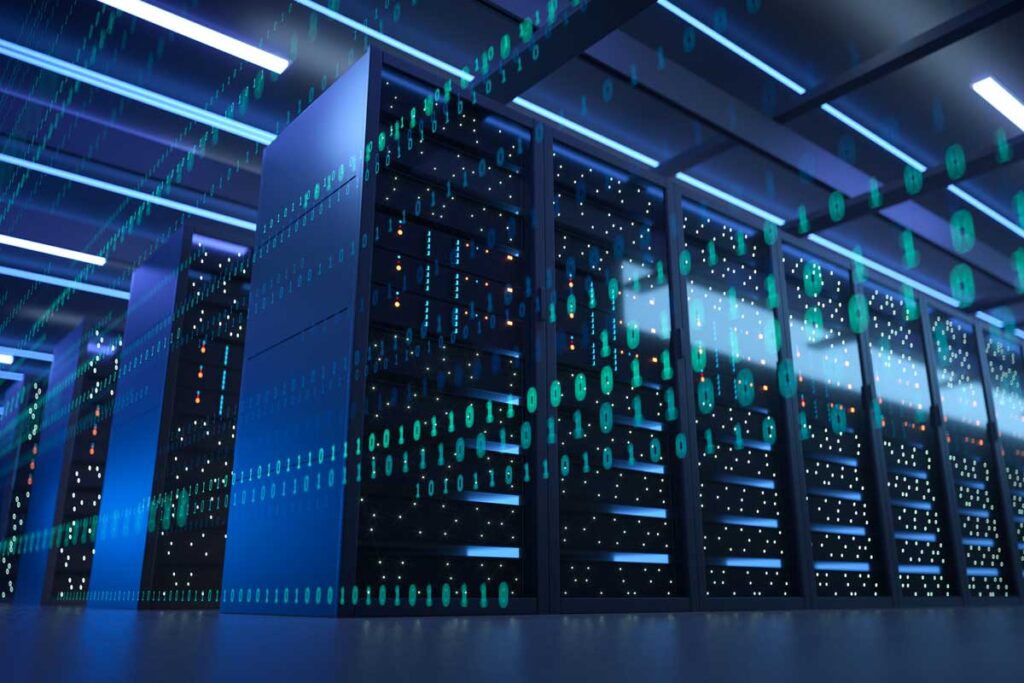
Course Content Overview
Day 1: Fundamentals of OpenMP Programming
- Session 1: Introduction to Parallel Computing and OpenMP Basics
- Overview of parallel computing concepts
- Introduction to shared memory architectures
- Session 2: Parallelizing Code with OpenMP Directives
- Basic OpenMP syntax and directives (`#pragma omp`)
- Parallel regions and thread creation
- Hands-on examples of basic parallelization
- Session 3: Work-Sharing Constructs in OpenMP
- `for` loops and task distribution among threads
- Sections and single directives for workload division
- Practical exercises on work-sharing constructs
- Session 4: Synchronization and Data Sharing in OpenMP
- Understanding shared vs. private variables
- Synchronization constructs: critical, atomic, barriers, etc.
- Avoiding race conditions and deadlocks
Day 2: Advanced Techniques and Optimization
- Session 1: Advanced Features of OpenMP
- Nested parallelism and dynamic thread management
- Tasking model in OpenMP (`task` and `taskwait`)
- Session 2: Performance Tuning and Load Balancing
- Profiling OpenMP applications for performance bottlenecks
- Scheduling policies: static, dynamic, guided scheduling
- Session 3: Debugging and Error Handling in OpenMP Programs
- Common debugging techniques for shared memory programs
- Tools for debugging (e.g., GDB) and runtime error detection
- Session 4: Case Studies and Best Practices in OpenMP Programming
- Real-world examples of OpenMP applications in various domains
- Writing scalable and maintainable code using best practices
Learning Outcomes
By the end of this course, participants will be able to:
- Understand the principles of shared memory parallelism and the role of OpenMP in multi-core systems.
- Write parallel programs using OpenMP directives for task distribution and synchronization.
- Identify and resolve common issues such as race conditions, deadlocks, and performance bottlenecks.
- Utilize advanced features like nested parallelism, tasking, and dynamic scheduling for complex applications.
- Optimize shared memory applications for improved performance while adhering to best practices in code design.