Course Description
NCC Monteengro developed a specialized training program to equip participants with foundational Python programming skills while integrating fundamentals of High-Performance Computing (HPC). Designed through consultations with industry experts and students, this course addresses the computational challenges faced in modern data-intensive applications. With an initial enrollment of over 40 students, the program emphasizes practical implementation and scalability, preparing participants for data-driven transformations in research and industry.
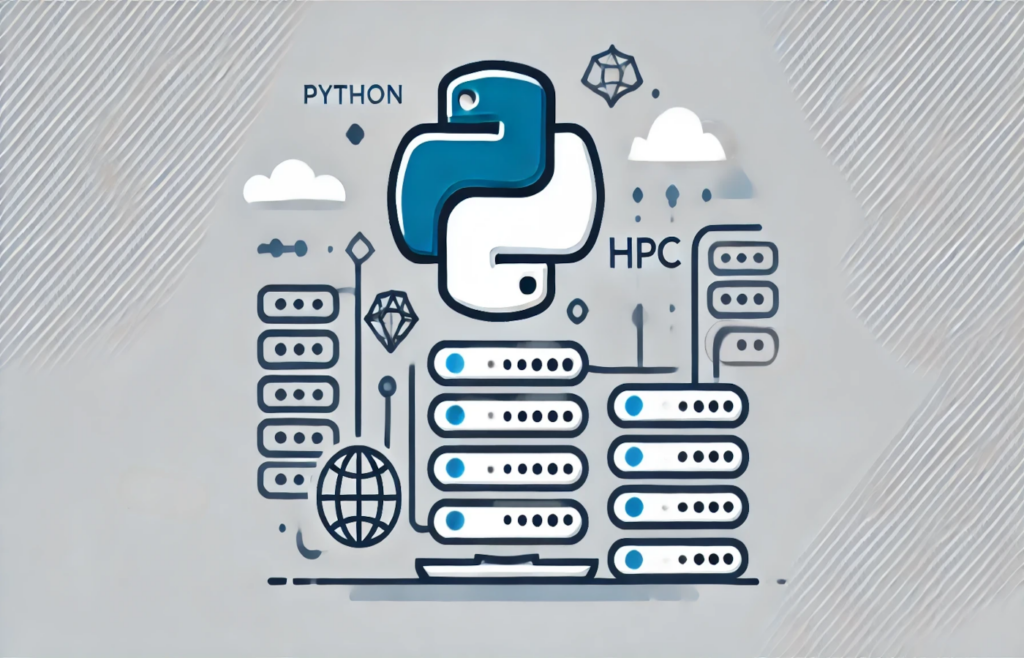
Python is a versatile and widely used programming language, making it a cornerstone for developing AI applications and data-intensive solutions. However, integrating Python with HPC concepts opens new possibilities for scalable computations and complex problem-solving. This course bridges theoretical principles and hands-on practices, enabling participants to design, implement, and optimize Python applications for HPC environments. Whether preparing for careers in AI research, engineering, or enterprise-level applications, participants will gain essential knowledge and hands-on experience. As industries increasingly rely on AI and large-scale data processing, this course prepares participants to tackle challenges in fields such as scientific computing, machine learning, and data analytics. By combining foundational programming skills with HPC techniques, students gain the ability to develop scalable solutions that drive digital transformation and innovation.
Course Content Overview (12 Modules)
- Introduction to Python Programming – Basic syntax, installation, and setting up the development environment.
- Data Types and Structures – Numbers, strings, Booleans, lists, tuples, sets, and dictionaries.
- Control Flow – Conditional statements, loops (for and while), and comprehension techniques.
- Functions and Modular Programming – Function creation, lambda expressions, and modular programming.
- File Handling – Reading, writing, and processing files including CSV and JSON formats.
- Object-Oriented Programming (OOP) – Classes, objects, inheritance, encapsulation, and polymorphism.
- Functional Programming – Using map, filter, reduce, and decorators for optimized workflows.
- Introduction to High-Performance Computing – Basics of HPC, parallel computing, and multiprocessing.
- Python Libraries for HPC – Using NumPy, Pandas, Numba
- Asynchronous Programming – Working with the asyncio library to handle concurrent tasks.
- Python simple HPC Project – Building a simple app with performance optimization using HPC concepts. From Google Colab to HPC.
- Finalization and Optimization – Debugging, profiling, and optimizing Python code for scalability.
Learning Outcomes
- Write and execute Python programs effectively.
- Apply fundamental programming principles, including data structures and control flow.
- Develop modular, object-oriented, and functional code.
- Implement file processing and data manipulation tasks.
- Understand and apply HPC concepts, including parallel and asynchronous programming.
- Utilize Python libraries for numerical computing and data analysis.
- Optimize Python applications for scalability in HPC environments.
- Build and enhance Python projects with computational performance improvements.